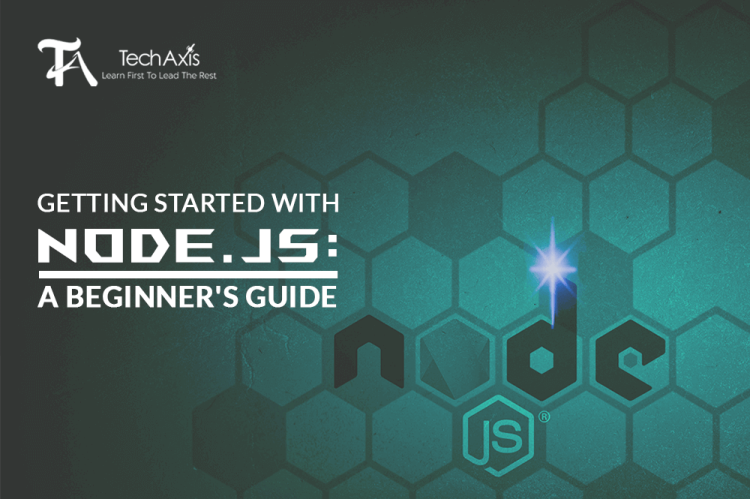
Getting Started with Node.js: A Beginner's Guide
In the world of web development, there are countless tools and technologies to choose from. But when it comes to building high-performance and scalable applications, one name stands out above the rest: Node.js.
Node js is an open-source, cross-platform JavaScript runtime environment and it has impressively revolutionized the way developers build web applications. It has made web development easier and faster than ever before.
Let’s dive into the world of Node.js and explore how you can use this powerful tool for building web applications. This beginner’s guide for Node.js will provide you with everything you need to know to get up and running with Node.js. Let's get started.
Basic Concepts of Node.js
Before we dive into the practical application of Node.js, it's essential to understand some basic concepts that form the foundation of the technology. Below are a few critical concepts that you need to grasp to become proficient in Node.js.
Asynchronous programming
Node.js is based on an event-driven, non-blocking I/O model that relies heavily on asynchronous programming. Asynchronous programming allows you to perform multiple tasks simultaneously and handle operations without blocking the execution of the main thread. With Node.js, you can perform I/O operations like reading and writing files or querying databases without waiting for a response.
Modules
Node.js is built on the CommonJS module system, which allows you to break your code into reusable modules. Modules in Node.js are essentially JavaScript files that expose some functionality using the module.exports object.
When you require a module in Node.js, it will load the file from the disk and execute its code, returning the module.exports object. This allows you to use the functionality of the module in your code.
Understanding Node.js Runtime
Node.js has a runtime that allows it to run JavaScript outside of the browser. The runtime is built on the V8 engine, which is the same engine that Google Chrome uses. The runtime provides a number of built-in modules that you can use to interact with the file system, network, and more.
Callback Functions
A callback function is a function passed as a parameter to another function. Callback functions are an essential part of Node.js and are used extensively to handle asynchronous operations. When a function completes its operation, it calls back the callback function with the results.
How to Install Node.js?
The installation process for Node.js is straightforward and can be done in a few simple steps.
Download the installer: Go to the official Node.js website (https://nodejs.org/) and download the installer for your operating system. Node.js is available for Windows, Mac, and Linux.
Run the installer: Once the installer has finished downloading, run it and follow the instructions to complete the installation process. You can choose to use the default settings or customize the installation to suit your needs.
Verify the installation: After the installation is complete, open your command prompt or terminal and type node -v. This should display the version of Node.js that you have installed. If you see a version number, then Node.js is successfully installed on your computer.
Congratulations! You have successfully installed Node.js on your computer.
Create Your First Node.js Application
Now that you have Node.js on your computer installed, you can start creating your first application. Open up your favorite code editor and create a new file called app.js. In the file, you will create a simple web server that listens for incoming requests and responds with a message.
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!');
});
server.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
Save the file and open up a terminal window. Navigate to the directory where you saved app.js, and run the following command:
node app.js
This will start the web server on port 3000. Open up a web browser and navigate to http://localhost:3000. You will see the message "Hello, World!" displayed in your browser.
NPM - Node Package Manager
Node.js comes with a built-in package manager called NPM (Node Package Manager). It’s a powerful tool that allows you to manage Node.js packages and dependencies easily. You can install, manage, and share packages (libraries, frameworks, and tools) with it in Node.js applications.
To install a package using NPM, simply run the following command in your terminal:
npm install
This will install the specified package and add it to your project's package.json file, which keeps track of all the dependencies for your project.
Express.js - A popular Node.js framework
Express.js is a popular framework for building web applications in Node.js. It provides a set of features for building web servers, handling HTTP requests, and creating APIs. Express.js is easy to use and can be integrated with other popular Node.js packages.
You can install Express.js using NPM:
npm install express
Once installed, you can create a new Express.js application by creating a new file and adding the following code:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, World!');
});
app.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
The above code will create an Express.js application that listens for incoming requests on port 3000. When a request is received at the root URL (/), it will respond with the message "Hello, World!".
Debugging Node.js applications
Debugging Node.js applications can be challenging, especially for beginners. Thankfully, Node.js comes with built-in debugging tools that make it easier to identify and fix issues in your code.
You can debug a Node.js application by running it in debug mode. Simply add the --inspect flag when running your application:
node --inspect app.js
This will start your application in debug mode and open a debugging port (usually 9229). You can then use a debugging tool like Chrome DevTools to connect to your application and start debugging.
So that was it. With all the knowledge we provided in this blog, you should be able to start building your own Node.js applications and exploring the vast ecosystem of Node.js packages and tools.